assert()
Functionality of assert() is very simple. It can be briefly described as follows.
The expression can be any C expression that gives on int result (usually comparision statement). If the expression gives non-negative value (i.e, value >= 0) the assert is doing nothing, but if the expression gives negative value it prints an error message in stderr (i.e, console) and abort(terminate) the program. The keyword here is 'abort(terminate) the program'.
It is usually used in debuging purpose and when you want to make it sure that a certain condition is met before the program to go any further.
It is handy function to use but suggest you to get clear understanding on how it works and why you want to use it. A few bullets below may help you with this.
- You can disable assert() by defining NDEBUG. This can be both handy and annoying depending on how you use it. It can be handy because you can just define NDEBUG definition if you don't want to use it assert() being scattered here and there in your code and put any performance overhead. It can be annoying if you misuse it. For example, if you do assert(x++) and you define NDEBUG, then x++ would not be evaluated because assert(x++) is not compiled at all. Don't put any expression
except comparision in assert() unless you know exactly what is going to happen.
Example 01 >
#include <assert.h> // you need to include assert.h to use assert() function.
#include <stdio.h>
int main()
{
int x;
x = -1;
assert(x >= 0); // the expression within assert() gives FALSE, so assert() will be triggered
printf("Hello World ! \n");
return 1;
}
|
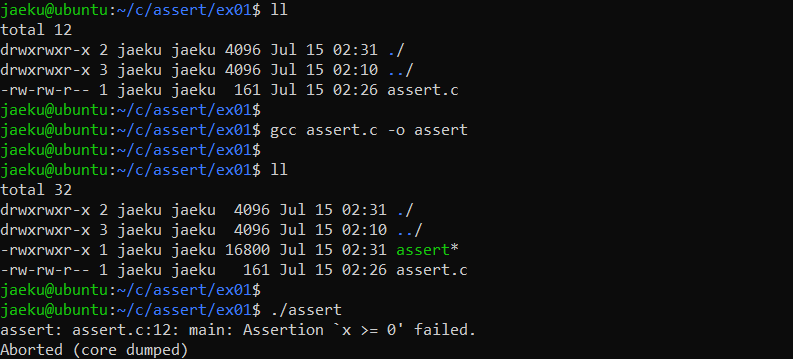
Example 02 >
#include <assert.h>
#include <stdio.h>
int main()
{
int x;
x = -1;
assert(x >= 0 && "x cannot be a negative value"); // you can put your own message in this way.
printf("Hello World ! \n");
return 1;
}
|
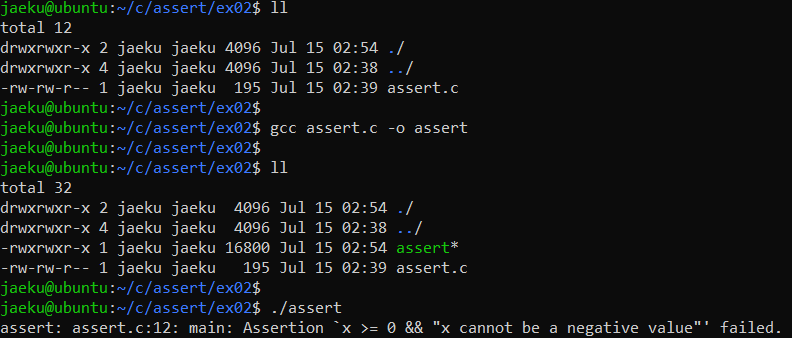
Example 03 >
#define NDEBUG // you can disable assert() by defining NDEBUG.
// You have the define this before #include <assert.h>
#include <assert.h>
#include <stdio.h>
int main()
{
int x;
x = -1;
assert(x >= 0 && "x cannot be a negative value");
printf("Hello World ! \n");
return 1;
}
|
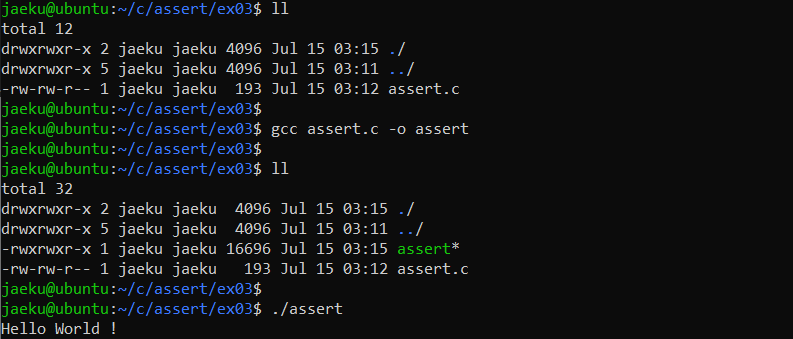
Reference :
|