static
You sould see the static keyword quite frequently used, but you may get confused sometimes since the meaning of the keywords would change depending on the context. Especially the meaning get different a lot depending on whether it is used with a variable or function.
The static keyword in C has several different uses and meanings, depending on the context in which it is used. Here are the main ways the static keyword is used in C:
- Static Variables: When a variable is declared as static within a function, it retains its value between function calls. Unlike local variables, which are destroyed when the function returns, static variables persist and maintain their value.
- Static Functions: When a function is declared as static, it can only be accessed within the same file (translation unit) in which it is defined. This allows you to keep certain functions "private" to a specific file.
- Static Global Variables: When a global variable is declared as static, it is only accessible within the file (translation unit) in which it is defined. This is useful for creating "private" global variables that are not exposed to the entire program.
- Static Allocation: The static keyword can also be used in the context of memory allocation. When a variable is declared without any storage class specifier (like auto or register), it is implicitly static. This means the variable is allocated storage in the static storage area, rather than the stack.
When the static keyword is used with variable, it mean 'preserve the result even when the variable gets out of the scope (e.g, out of the function that defines the variable).
It would be easier to understand if you take a look at the examples.
Example 01 >
This example shows the difference in behavior between local variables and static variables in C. Here's a high-level description of what the code does:
The staticIncrement() function is defined, which contains two variables: a and b.
- a is a regular local variable, which means it is allocated on the stack and its value is reset to 0 every time the function is called.
- b is a static variable, which means it is allocated in the static memory area and retains its value between function calls.
Inside the staticIncrement() function:
- It prints a separator line to indicate the function is being called.
- It prints the initial values of a and b.
- It increments the values of a and b.
- It prints the updated values of a and b.
The main() function is defined, which calls the staticIncrement() function five times.
Each time staticIncrement() is called:
- The local variable a is reset to 0 and its value is incremented.
- The static variable b retains its value from the previous call and is incremented.
The key point of this code is to demonstrate the difference between local variables and static variables. Local variables are allocated on the stack and their values are reset on each function call, while static variables are allocated in the static memory area and retain their values between function calls.ute to environmental monitoring and sustainability efforts?
#include <stdio.h>
#include <string.h>
void staticIncrement()
{
int a = 0; // Pay attention to how the value for this variable(not static) changes
static int b = 0; // Pay attention to how the value for this variable(static) changes
printf("staticIncrement() is called -----------------------------\n");
printf(" Before Increment : a = %d, b = %d\n",a,b) ;
a++;
b++;
printf(" After Increment : a = %d, b = %d\n",a,b) ;
}
int main()
{
staticIncrement();//at the end of the this call,'a' is removed but b stays there with the result
staticIncrement();//at the end of the this call,'a' is removed but b stays there with the result
staticIncrement();//at the end of the this call,'a' is removed but b stays there with the result
staticIncrement();//at the end of the this call,'a' is removed but b stays there with the result
staticIncrement();//at the end of the this call,'a' is removed but b stays there with the result
return 1;
}
|
Result :
In this result, you would notice that the variable 'a' get re-initialized at the every function call. So the result are the same for every function call. But the variable 'b' (static variable) does not disappear and remain with the result so the value changes (increments) by one at every function call.
staticIncrement() is called ----------------------------- Before Increment : a = 0, b = 0 After Increment : a = 1, b = 1
staticIncrement() is called ----------------------------- Before Increment : a = 0, b = 1 After Increment : a = 1, b = 2
staticIncrement() is called ----------------------------- Before Increment : a = 0, b = 2 After Increment : a = 1, b = 3
staticIncrement() is called ----------------------------- Before Increment : a = 0, b = 3 After Increment : a = 1, b = 4
staticIncrement() is called ----------------------------- Before Increment : a = 0, b = 4 After Increment : a = 1, b = 5
|
When the static keyword is used with the function, it defines the scope of the function. Basically the function definition has global scope, but when it is defined with the static, it makes the function local which is visiable only in the c file that defines the function.
For this as well, it will be clearer if you take a look at examples.
Example 01 >
In this example file has the definition of the function print_hello() without static keyword meaning that this function would have global scope. Compare this with Example 02.
file1.c |
file2.c |
void print_hello();
int main(void)
{
print_hello();
return 0;
}
|
#include <stdio.h>
void print_hello()
{
printf("Hello World\n");
}
|
It compiles and runs without any problem since the print_hello() in file2.c is visiable from file1.c as well.
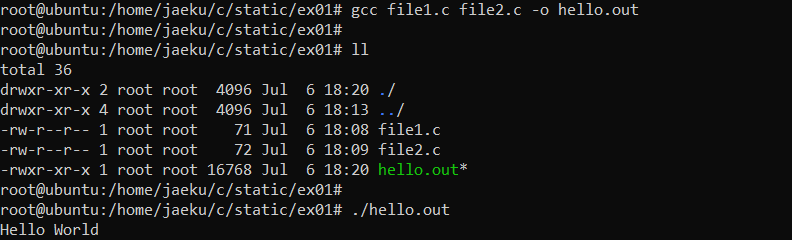
Example 02 >
This example is almost same as example 1 except the 'static' keyword is used. As you see here, print_hello() is defined as a static function in file2.c. It mean that the function is local to file2.c
This example demonstrates the use of the static keyword to make a function local to a specific file, and the consequences of having a function with the same name but different definitions across multiple files.
- The code consists of two files: file1.c and file2.c.
- In file1.c, there is a function called print_hello() that is defined as static. This means the function is local to the file1.c file and cannot be accessed from other files.
- In file2.c, there is also a function called print_hello(), but it is not defined as static. This means the function is global and can be accessed from other files.
- When the code is compiled, the linker cannot find the definition of the print_hello() function in file1.c because it is a static function and is not visible outside of that file. This results in a linker error.
file1.c |
file2.c |
static void print_hello();
int main(void)
{
print_hello();
return 0;
}
|
#include <stdio.h>
static void print_hello()
{
printf("Hello World\n");
}
|
Now let's try to compile and see what happens. As you see, you would get the error as shown below. this is because the function definition in file2.c is not visible to file1.c.

Example 03 >
In this example, I have defined the same function name (the contents of the function is different) in both file 1.c and file2.c as non static functions. It means that both function has global scope. Compare this with example 04.
file1.c |
file2.c |
void print_hello()
{
printf("Hello World in file1\n");
}
int main(void)
{
print_hello();
return 0;
}
|
#include <stdio.h>
void print_hello()
{
printf("Hello World in file2\n");
}
|
Now let's compile and see what happens. You get this error because you have two functions with the same function in the same scope (global scope).

Example 04 >
Now let's modify the example 03 as below. I just put the static keyword to both of the functions in file1.c and file2.c. Now the print_hello() function in file2.c is visible only in file2 and the print_hello() function in file1.c is visiable only in file1.c.
This example shows the use of the static keyword to control the visibility and accessibility of functions across multiple C files.
The key points are:
- file1.c and file2.c both contain a function called print_hello().
- In file1.c, the print_hello() function is defined as static, which means it is local to that file and cannot be accessed from other files.
- In file2.c, the print_hello() function is also defined as static, which means it is local to that file and cannot be accessed from other files.
- When the code is compiled and executed, the output shows that the print_hello() function in file1.c is called, and the print_hello() function in file2.c is also called, but they are isolated from each other due to the static keyword.
This example showcases the use of the static keyword to create private functions within a specific file, preventing name collisions and ensuring encapsulation of functionality. By making the print_hello() functions static, they are effectively hidden from the rest of the program, and the only way to call them is from within their respective files.
This approach helps maintain modularity and organization in larger C projects, where you want to prevent unintended interactions between different parts of the codebase.
file1.c |
file2.c |
static void print_hello()
{
printf("Hello World in file1\n");
}
int main(void)
{
print_hello();
return 0;
}
|
#include <stdio.h>
static void print_hello()
{
printf("Hello World in file2\n");
}
|
Now it compiles and runs without any problem as shown below.
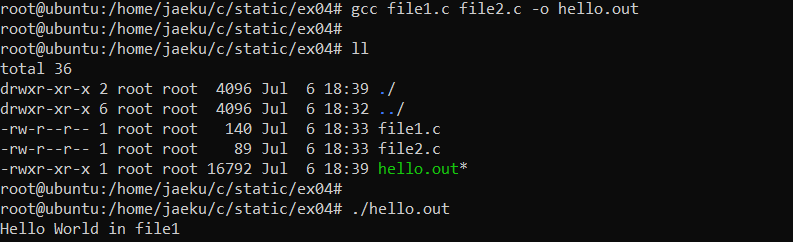
Example 05 >
Now let's modify the example 04 as below. I just removed the static keywords from both files.
This example shows the consequences of removing the static keyword from the print_hello() function definitions in both file1.c and file2.c.
The key points are:
In the modified code, the static keyword has been removed from both print_hello() functions, making them global functions.
- When the program is compiled, the linker encounters a problem. There are now two global print_hello() functions defined, one in file1.c and one in file2.c.
- The linker is unable to determine which print_hello() function to use when called from the main() function in file1.c. This results in a linker error, as indicated by the "multiple definition of 'print_hello'" message.
- The program cannot be compiled and executed successfully due to this linker error, which is caused by the naming conflict between the two global print_hello() functions.
This example illustrates the importance of using the static keyword to maintain modularity and prevent naming conflicts in C programs. By removing the static keyword, the print_hello() functions become global, which can lead to linker errors when there are multiple definitions of the same function name across different files.
The static keyword is a crucial tool for controlling the scope and visibility of functions and variables in C, helping to ensure that the program's components are properly isolated and can be developed and maintained independently.
file1.c |
file2.c |
#include <stdio.h>
void print_hello()
{
printf("Hello World in file1\n");
}
int main(void)
{
print_hello();
return 0;
}
|
#include <stdio.h>
void print_hello()
{
printf("Hello World in file2\n");
}
|

Reference :
|
|