API
There are may ways to test the mongoDB API. I will show you a few most basic ways to test the API. I don't think you would use these ways in your development, but these methods can be a quick and easy way to check if the API is working properly.
Test with Postman
Postman is a popular API development tool that also includes functionality for testing APIs. With Postman, you can easily create and send HTTP requests to your API, inspect the responses, and validate the results
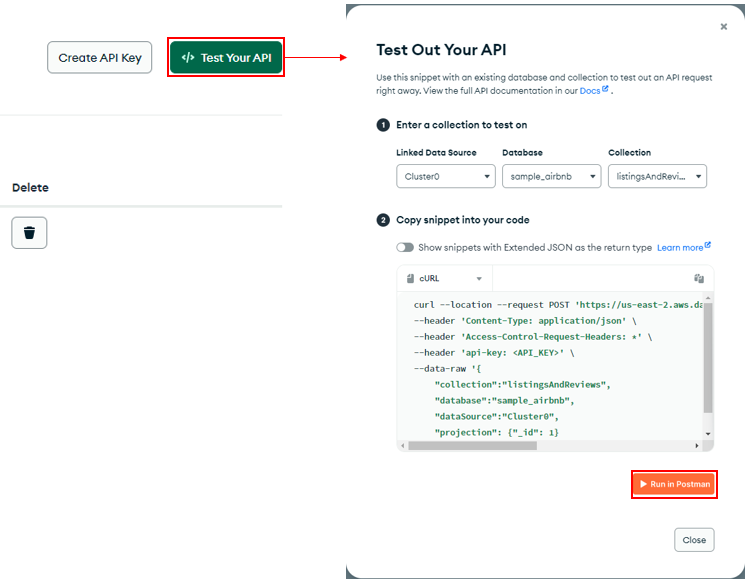
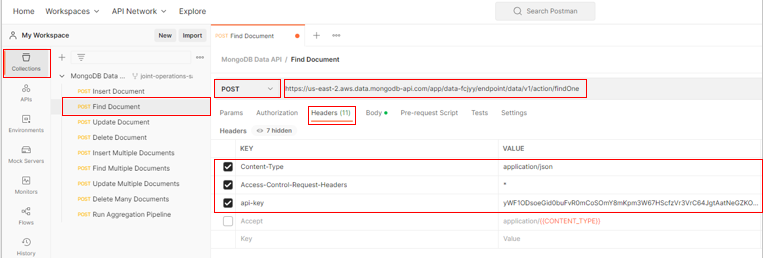
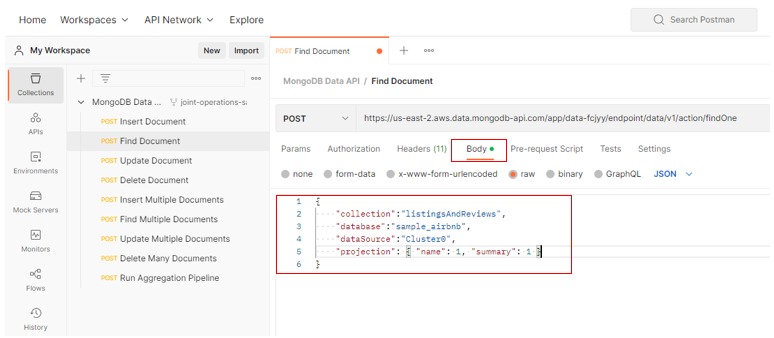
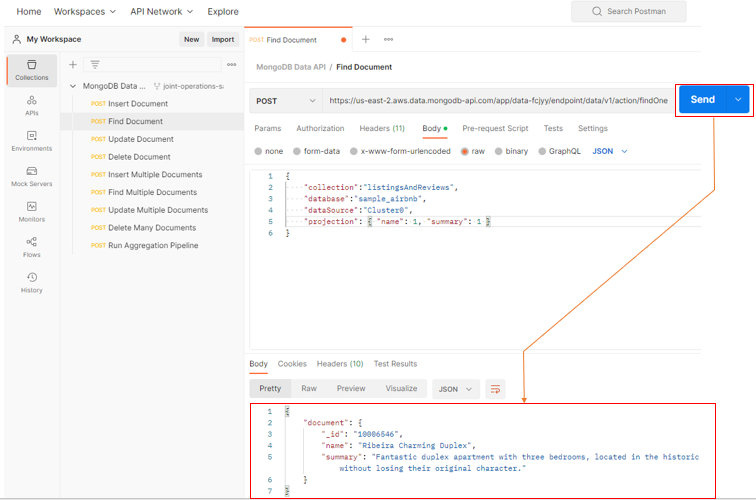
Test with cURL
Connection Test
Input (Linux)
|
jk@jk-VirtualBox:~$ curl --location --request POST 'https://us-east-2.aws.data.mongodb-api.com/app/data-fcjyy/endpoint/data/v1/action/findOne' \
--header 'Content-Type: application/json' \
--header 'Access-Control-Request-Headers: *' \
--header 'api-key:<API_KEY>' \
--data-raw '{
"collection":"listingsAndReviews",
"database":"sample_airbnb",
"dataSource":"Cluster0",
"projection": {"_id": 1}
}'
|
Input (Windows 11 PowerShell)
|
C:\> Invoke-RestMethod -Method POST -Uri 'https://us-east-2.aws.data.mongodb-api.com/app/data-fcjyy/endpoint/data/v1/action/findOne' `
-Headers @{
'Content-Type' = 'application/json'
'Access-Control-Request-Headers' = '*'
'api-key' = '<API_KEY>'
} -Body '{
"collection":"listingsAndReviews",
"database":"sample_airbnb",
"dataSource":"Cluster0",
"projection": {"_id": 1}
}'
|
Result
|
{"document":{"_id":"10006546"}}
|
Output Collection
Input
|
PS C:\> Invoke-RestMethod -Method POST -Uri 'https://us-east-2.aws.data.mongodb-api.com/app/data-fcjyy/endpoint/data/v1/action/find' `
-Headers @{
'Content-Type' = 'application/json'
'Access-Control-Request-Headers' = '*'
'api-key' = '<API_KEY>'
} -Body '{
"collection":"listingsAndReviews",
"database":"sample_airbnb",
"dataSource":"Cluster0",
"filter": {},
"projection": {},
"limit": 1
}'
|
Result
|
documents
---------
{@{_id=10006546; listing_url=https://www.airbnb.com/rooms/10006546; name=Ribeira Charming Duplex; summary=Fantastic ...
|
Input
|
PS C:\ Invoke-RestMethod -Method POST -Uri 'https://us-east-2.aws.data.mongodb-api.com/app/data-fcjyy/endpoint/data/v1/action/find' `
-Headers @{
'Content-Type' = 'application/json'
'Access-Control-Request-Headers' = '*'
'api-key' = '<API_KEY>'
} -Body '{
"collection":"listingsAndReviews",
"database":"sample_airbnb",
"dataSource":"Cluster0",
"filter": {},
"projection": {},
"limit": 1
}' | ConvertTo-Json
|
Input
|
jk@jk-VirtualBox:~$ curl -X POST 'https://us-east-2.aws.data.mongodb-api.com/app/data-fcjyy/endpoint/data/v1/action/find'
-H 'Content-Type: application/json'
-H 'Access-Control-Request-Headers: *'
-H 'api-key: <API_KEY>'
-d '{
"collection":"listingsAndReviews",
"database":"sample_airbnb",
"dataSource":"Cluster0",
"filter": {},
"projection": {},
"limit": 1
}'
|
Result
|
{
"documents": [
{
"_id": "10006546",
"listing_url": "https://www.airbnb.com/rooms/10006546",
"name": "Ribeira Charming Duplex",
"summary": "Fantastic duplex apartment with three bedrooms, located in ...",
"space": "Privileged views of the Douro River and Ribeira square,.....",
"notes": "Lose yourself in the narrow streets and staircases zone....",
"transit": "Transport: ⢠Metro station and S. Bento railway 5min; ....",
"access": "We are always available to help guests. The house is ...",
"interaction": "Cot - 10 ⬠/ night Dog - ⬠7,5 / night",
"house_rules": "Make the house your home...",
"property_type": "House",
"room_type": "Entire home/apt",
"bed_type": "Real Bed",
"minimum_nights": "2",
"maximum_nights": "30",
"cancellation_policy": "moderate",
"last_scraped": "2019-02-16T05:00:00Z",
"calendar_last_scraped": "2019-02-16T05:00:00Z",
"first_review": "2016-01-03T05:00:00Z",
"last_review": "2019-01-20T05:00:00Z",
"accommodates": 8,
"bedrooms": 3,
"beds": 5,
"number_of_reviews": 51,
"bathrooms": "1.0",
"amenities": "TV Cable TV Wifi Kitchen Paid parking off premises Smoking allowed ...",
"price": "80.00",
"security_deposit": "200.00",
"cleaning_fee": "35.00",
"extra_people": "15.00",
"guests_included": "6",
"images": "@{thumbnail_url=; medium_url=; picture_url=https://a0.muscache.com/im/pictures/..}",
"host": "@{host_id=51399391; host_url=https://www.airbnb.com/users/show/51399391;...",
"address": "@{street=Porto, Porto, Portugal; suburb=; government_area=Cedofeita, Ildefonso,..",
"availability": "@{availability_30=28; availability_60=47; availability_90=74; availability_365=239}",
"review_scores": "@{review_scores_accuracy=9; review_scores_cleanliness=9;...}",
"reviews": " "
}
]
}
|
Finding Matching Data
Input
|
C:\> Invoke-RestMethod -Method POST -Uri 'https://us-east-2.aws.data.mongodb-api.com/app/data-fcjyy/endpoint/data/v1/action/find' `
-Headers @{
'Content-Type' = 'application/json'
'Access-Control-Request-Headers' = '*'
'api-key' = '<API_KEY>'
} -Body '{
"collection":"listingsAndReviews",
"database":"sample_airbnb",
"dataSource":"Cluster0",
"filter": {},
"projection": { "name": 1, "summary": 1 },
"limit": 1
}' | ConvertTo-Json
|
Input
|
jk@jk-VirtualBox:~$ curl -X POST 'https://us-east-2.aws.data.mongodb-api.com/app/data-fcjyy/endpoint/data/v1/action/find'
-H 'Content-Type: application/json'
-H 'Access-Control-Request-Headers: *'
-H 'api-key: <API_KEY>'
-d '{
"collection":"listingsAndReviews",
"database":"sample_airbnb",
"dataSource":"Cluster0",
"filter": {},
"projection": {"name": 1, "summary": 1},
"limit": 1
}'
|
Result
|
{
"documents": [
{
"_id": "10006546",
"name": "Ribeira Charming Duplex",
"summary": "Fantastic duplex apartment with three bedrooms, located ..."
}
]
}
|
Input
|
C:\> Invoke-RestMethod -Method POST -Uri 'https://us-east-2.aws.data.mongodb-api.com/app/data-fcjyy/endpoint/data/v1/action/find' `
-Headers @{
'Content-Type' = 'application/json'
'Access-Control-Request-Headers' = '*'
'api-key' = '<API_KEY>'
} -Body '{
"collection":"listingsAndReviews",
"database":"sample_airbnb",
"dataSource":"Cluster0",
"filter": {},
"projection": { "name": 1, "summary": 1 },
"limit": 3
}' | ConvertTo-Json
|
Input
|
jk@jk-VirtualBox:~$ curl -X POST 'https://us-east-2.aws.data.mongodb-api.com/app/data-fcjyy/endpoint/data/v1/action/find'
-H 'Content-Type: application/json'
-H 'Access-Control-Request-Headers: *'
-H 'api-key: <API_KEY>'
-d '{
"collection":"listingsAndReviews",
"database":"sample_airbnb",
"dataSource":"Cluster0",
"filter": {},
"projection": {"name": 1, "summary": 1},
"limit": 3
}'
|
Result
|
{
"documents": [
{
"_id": "10006546",
"name": "Ribeira Charming Duplex",
"summary": "Fantastic duplex apartment with three bedrooms, located in the historic area of ..."
},
{
"_id": "10009999",
"name": "Horto flat with small garden",
"summary": "One bedroom + sofa-bed in quiet and bucolic neighbourhood right next to ..."
},
{
"_id": "1001265",
"name": "Ocean View Waikiki Marina w/prkg",
"summary": "A short distance from Honolulu\u0027s billion dollar mall, and the same distance ..."
}
]
}
|
|
|