Regular Expression
this code is created first by chatGPT on Jan 17 2023 (meaning using chatGPT 3.5) and then modified a little bit my me. The initial request that I put into chatGPT is as follows :
Write a javascript with the following requirement
1. create a table with width = 600 at the center of the page
2. the table has three rows and one column, make grid visible in thin line
3. put a text field that can take in a long string. Put some good string suitable for Regular Expression testing
4. In the second row, put a text box with the name = 'RegString' at the left, a drop downbox of name = 'RegOperator' with the elements of test,exec,match,search,replace,split at the center, a button labeled as 'Process'.
5. In the third row, put a text field with the name = 'Result'
6. Write a javascript that apply the RegOperator to the string in RegString and output the result in 'Result' text field8. javascript for the plot should be stored in a separate file
7. Create javascript and html in separate file
|
NOTE : It is not guaranteed that you would have the same code as I got since chatGPT produce the answers differently depending on the context. And it may produce the different answers everytime you ask even with the exact the same question.
NOTE : If you don't have any of your own idea for the request, copy my request and paste it into the chatGPT and put additional requests based on the output for the previous request. I would suggest to create a new thread in the chatGPT and put my request and then continue to add your own request.
RegularExpression.html
|
<html>
<head>
</head>
<body>
<table style="width: 600px; margin: 0 auto; border-collapse: collapse;">
<tr>
<td>
<textarea id="inputString" style="width: 100%;">This is a good string suitable for Regular Expression testing</textarea>
</td>
</tr>
<tr>
<td>
<input type="text" id="RegString" value="[A-Za-z]+" style="width: 70%;" placeholder="Enter regular expression here" >
<select id="RegOperator">
<option value="test">test</option>
<option value="exec">exec</option>
<option value="match">match</option>
<option value="search">search</option>
<option value="replace">replace</option>
<option value="split">split</option>
</select>
<button onclick="process()">Process</button>
</td>
</tr>
<tr>
<td>
<textarea id="Result" style="width: 100%;"></textarea>
</td>
</tr>
</table>
<script src="RegularExpression.js"></script>
</body>
</html>
|
RegularExpression.js
|
function process() {
let inputString = document.getElementById("inputString").value;
let RegString = document.getElementById("RegString").value;
console.log(RegString);
let regex = new RegExp(RegString.replace(/'/g,'').replace(/\//g,''),'g');
console.log(regex);
let RegOperator = document.getElementById("RegOperator").value;
let result = "";
switch(RegOperator) {
case "test":
result = regex.test(inputString);
result = result.toString();
break;
case "exec":
result = regex.exec(inputString);
console.log(result);
break;
case "match":
result = inputString.match(regex);
console.log(result);
break;
case "search":
result = inputString.search(regex);
result = result.toString();
break;
case "replace":
result = inputString.replace(regex, "replacement string");
break;
case "split":
result = inputString.split(regex);
console.log(result);
break;
default:
result = "Invalid operator";
}
document.getElementById("Result").value = result;
}
|
NOTE : regex is supposed to have Regular Expression, but if I just pass the contents of RegString to RegExp() it just passing the regular text (i.e, the value of a text field). This caused problem. To workaround this problem and finally reach the solution new RegExp(RegString.replace(/'/g,'').replace(/\//g,''),'g'), I spent over an hour chatting with chatGPT. Even though chatGPT did not write the correct code at first, it helped me a lot with the debugging process.
NOTE : console.log() is added for debugging purpose. You will not see the result of console.log() in web page. In order to see the result of console.log(), you need to run Development Tool. The way to open up the tool in Google Chrome is shown below.
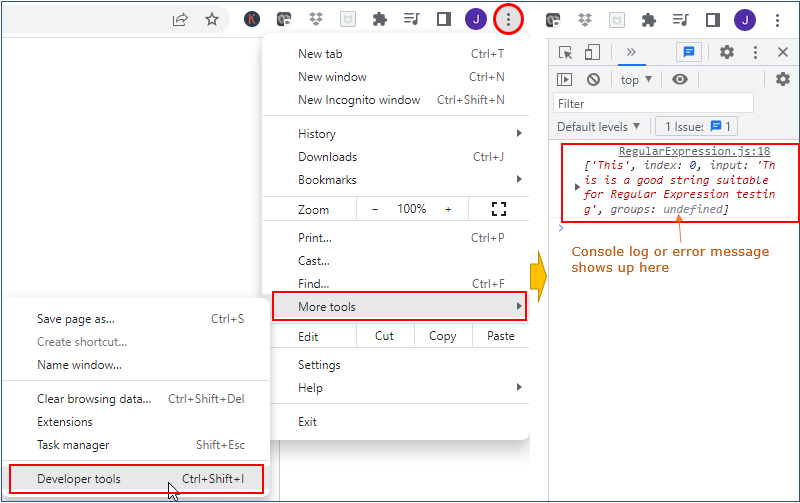
|
|