Basic Circuit with Qiskit
In this note, I will create create the same circuit as the one created by Quantum Composer in this note. In case of very simple circuit, it would be easiler and intuitive to create them with Composer, but at some point you may need to use scripting. The purpose of this note is to get familiar with basic syntax of Qiskit and
basic script structure to build and process the result.
Summary of the basic circuits
Followings are short summary of basic circuit components which are most frequently used :
- Pauli Gates (X, Y, Z):
- X Gate (Pauli-X, NOT gate): Flips the state of a qubit (|0> to |1> and vice versa). Used in many quantum algorithms for state inversion.
- Y Gate (Pauli-Y): A more complex rotation that affects both the real and imaginary components of a qubit's state.
- Z Gate (Pauli-Z, Phase-flip gate): Changes the phase of a qubit without changing its amplitude.
- Hadamard Gate (H):
- Creates a superposition of |0> and |1> from a definite state. It's fundamental in algorithms like Grover's and in creating entangled states.
- Phase Shift Gates (S, T):
- S Gate (πi/4 gate): Adds a phase of πi/2. Used for manipulating the phase of qubits.
- T Gate (πi/8 gate): Applies a πi/4 phase shift. It's important for more precise qubit phase manipulation.
- Controlled Gates (CNOT, CZ, etc.):
- CNOT Gate (Controlled-NOT): Entangles two qubits, flipping the second (target) qubit if the first (control) qubit is |1>. Essential for quantum teleportation and superdense coding.
- CZ Gate (Controlled-Z): Another form of controlled phase flip. Useful in creating various quantum states and in quantum error correction.
- Swap Gate:
- Swaps the states of two qubits. Useful in quantum algorithms where qubit position is relevant.
- Toffoli Gate (CCNOT):
- A three-qubit gate that performs a NOT on the third qubit only when the first two qubits are in the |1⟩ state. Key in quantum error correction and reversible computing.
- Fredkin Gate (Controlled-SWAP):
- A three-qubit gate that swaps the last two qubits if the first qubit is |1⟩. Used in quantum computing for operations that require conditional logic.
Basic Script Template
The Qiskit script used in this note are mostly the same structure that is made up of several blocks as below.
- Importing the necessary packets
- Build a Circuit
- Setup a circuit (create registers)
- Define circuit operations
- Visualize(draw) the circuit
- Run the circuit
- Simulate the circuit
- Visualize the result
Following code is a simple example based on this structure
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
from qiskit.visualization import plot_histogram
from numpy import pi
qreg_q = QuantumRegister(4, 'q')
creg_c = ClassicalRegister(4, 'c')
circuit = QuantumCircuit(qreg_q, creg_c)
circuit.h(qreg_q[0])
circuit.cx(qreg_q[0], qreg_q[1])
circuit.measure(qreg_q[0], creg_c[0])
circuit.measure(qreg_q[1], creg_c[1])
circuit.draw('mpl')
simulator = Aer.get_backend('qasm_simulator')
job = execute(circuit, simulator, shots=10000)
result = job.result()
counts = result.get_counts(circuit)
plot_histogram(counts)
|
Now let's break down the code line by line
- Importing the necessary packets
- from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer: This line imports necessary components from Qiskit.
- QuantumRegister and ClassicalRegister are for creating quantum and classical registers, respectively.
- QuantumCircuit is used to build quantum circuits. execute runs the circuits,
- Aer is a module for simulating quantum circuits.
- from qiskit.visualization import plot_histogram: This imports the plot_histogram function from Qiskit, which is used to visualize the results of quantum experiments.
- from numpy import pi: Imports the constant pi from the Numpy library, useful for mathematical operations, particularly those involving angles in quantum gates.
- Build a Circuit
- qreg_q = QuantumRegister(4, 'q'): Creates a quantum register named 'q' with 4 qubits.
- creg_c = ClassicalRegister(4, 'c'): Creates a classical register named 'c' with 4 bits.
- circuit = QuantumCircuit(qreg_q, creg_c): Initializes a quantum circuit with the previously defined quantum and classical registers.
- circuit.h(qreg_q[0]): Applies the Hadamard gate to the first qubit in the quantum register. This gate puts the qubit into a superposition of states.
- circuit.cx(qreg_q[0], qreg_q[1]): Applies a Controlled-NOT (CNOT) gate with the first qubit as the control and the second qubit as the target. This gate entangles the two qubits.
- circuit.measure(qreg_q[0], creg_c[0]): Measures the first qubit and stores the result in the first bit of the classical register.
- circuit.measure(qreg_q[1], creg_c[1]): Measures the second qubit and stores the result in the second bit of the classical register.
- circuit.draw('mpl'): Draws the quantum circuit using Matplotlib ('mpl') for visualization.
- Run the circuit
- simulator = Aer.get_backend('qasm_simulator'): Retrieves the QASM (Quantum Assembly Language) simulator backend from Aer for simulation.
- job = execute(circuit, simulator, shots=10000): Executes the quantum circuit on the simulator for 10,000 runs ('shots').
- result = job.result(): Retrieves the result from the execution.
- counts = result.get_counts(circuit): Gets the count of each quantum state observed over all the shots.
- plot_histogram(counts): Plots a histogram of the counts, visualizing the probabilities of the quantum states.
H-CNOT with |0> and |0>
This example is for verification of following simple circuit : H(Hadamard) gate and CNOT gate connected in series as shown below. This circuit get two Qbits involved and I assume that the two bits getting into this circuit is |0> and |0>.
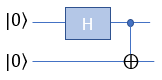
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
from qiskit.visualization import plot_histogram
from numpy import pi
# Existing circuit setup
qreg_q = QuantumRegister(4, 'q')
creg_c = ClassicalRegister(4, 'c')
circuit = QuantumCircuit(qreg_q, creg_c)
# Existing circuit operations
circuit.h(qreg_q[0])
circuit.cx(qreg_q[0], qreg_q[1])
circuit.measure(qreg_q[0], creg_c[0])
circuit.measure(qreg_q[1], creg_c[1])
circuit.draw('mpl')
|
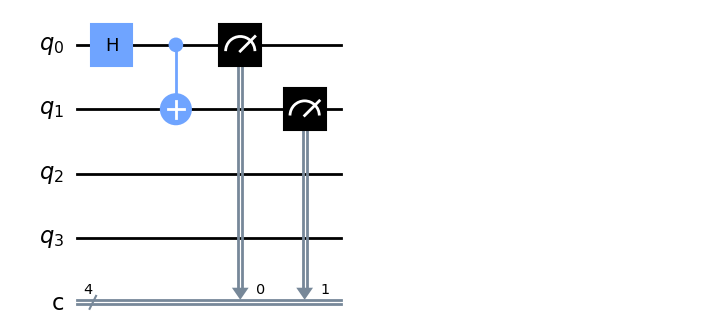
|
# Simulate the circuit
simulator = Aer.get_backend('qasm_simulator')
job = execute(circuit, simulator, shots=10000)
result = job.result()
# Get counts and plot the histogram
counts = result.get_counts(circuit)
plot_histogram(counts)
|
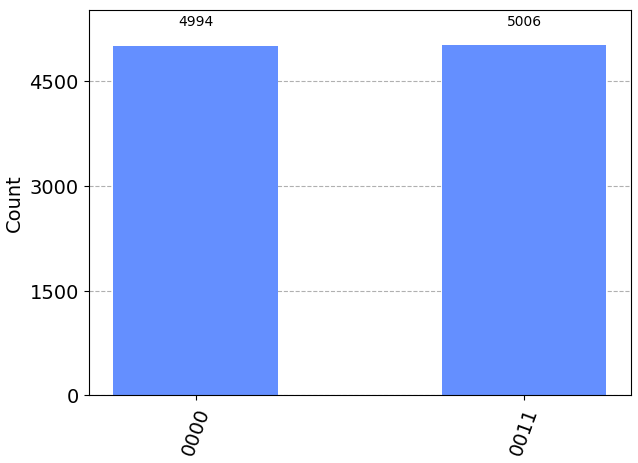
|
H-CNOT with |0> and |1>
This example is for verification of following simple circuit : H(Hadamard) gate and CNOT gate connected in series as shown below. This circuit get two Qbits involved and I assume that the two bits getting into this circuit is |0> and |1>
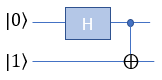
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
from qiskit.visualization import plot_histogram
from numpy import pi
# Existing circuit setup
qreg_q = QuantumRegister(4, 'q')
creg_c = ClassicalRegister(4, 'c')
circuit = QuantumCircuit(qreg_q, creg_c)
# Existing circuit operations
circuit.h(qreg_q[0])
circuit.x(qreg_q[1])
circuit.cx(qreg_q[0], qreg_q[1])
circuit.measure(qreg_q[0], creg_c[0])
circuit.measure(qreg_q[1], creg_c[1])
circuit.draw('mpl')
|
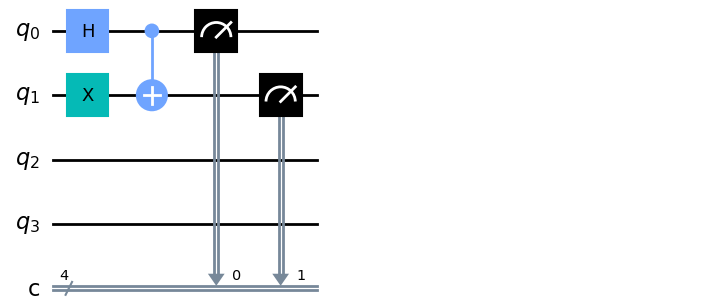
|
# Simulate the circuit
simulator = Aer.get_backend('qasm_simulator')
job = execute(circuit, simulator, shots=10000)
result = job.result()
# Get counts and plot the histogram
counts = result.get_counts(circuit)
plot_histogram(counts)
|
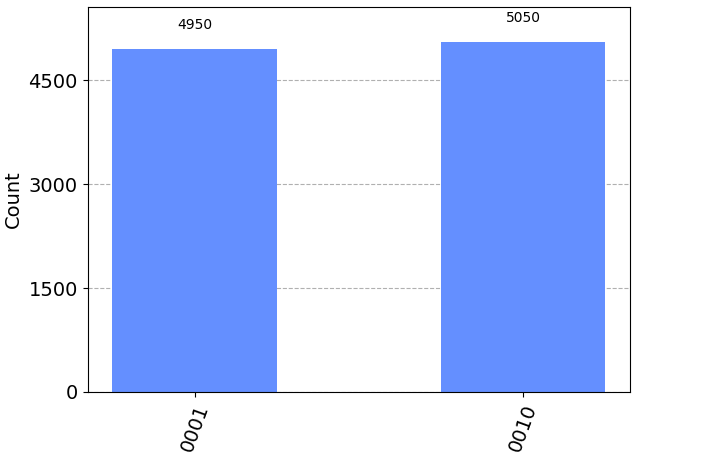
|
H-CNOT with |1> and |0>
This example is for verification of following simple circuit : H(Hadamard) gate and CNOT gate connected in series as shown below. This circuit get two Qbits involved and I assume that the two bits getting into this circuit is |1> and |0>
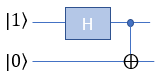
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
from qiskit.visualization import plot_histogram
from numpy import pi
# Existing circuit setup
qreg_q = QuantumRegister(4, 'q')
creg_c = ClassicalRegister(4, 'c')
circuit = QuantumCircuit(qreg_q, creg_c)
# Existing circuit operations
circuit.x(qreg_q[0])
circuit.h(qreg_q[0])
circuit.cx(qreg_q[0], qreg_q[1])
circuit.measure(qreg_q[0], creg_c[0])
circuit.measure(qreg_q[1], creg_c[1])
circuit.draw('mpl')
|
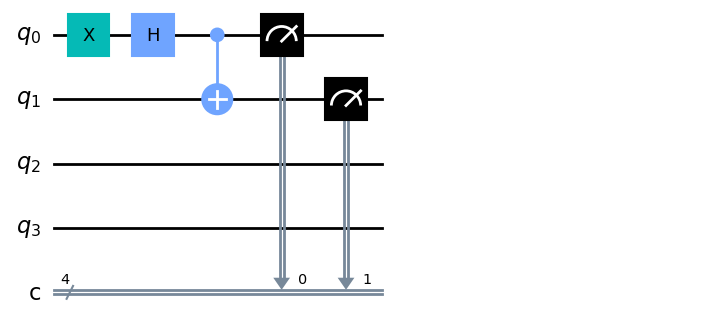
|
# Simulate the circuit
simulator = Aer.get_backend('qasm_simulator')
job = execute(circuit, simulator, shots=10000)
result = job.result()
# Get counts and plot the histogram
counts = result.get_counts(circuit)
plot_histogram(counts)
|
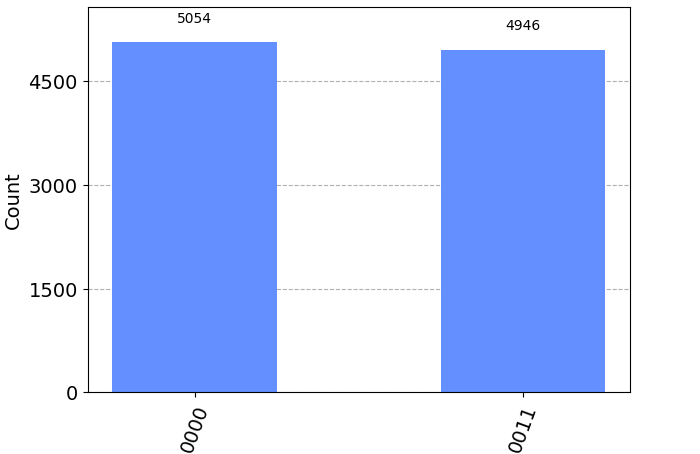
|
H-CNOT with |1> and |1>
This example is for verification of following simple circuit : H(Hadamard) gate and CNOT gate connected in series as shown below. This circuit get two Qbits involved and I assume that the two bits getting into this circuit is |1> and |1>
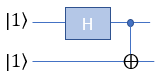
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
from qiskit.visualization import plot_histogram
from numpy import pi
# Existing circuit setup
qreg_q = QuantumRegister(4, 'q')
creg_c = ClassicalRegister(4, 'c')
circuit = QuantumCircuit(qreg_q, creg_c)
# Existing circuit operations
circuit.x(qreg_q[0])
circuit.h(qreg_q[0])
circuit.x(qreg_q[1])
circuit.cx(qreg_q[0], qreg_q[1])
circuit.measure(qreg_q[0], creg_c[0])
circuit.measure(qreg_q[1], creg_c[1])
circuit.draw('mpl')
|
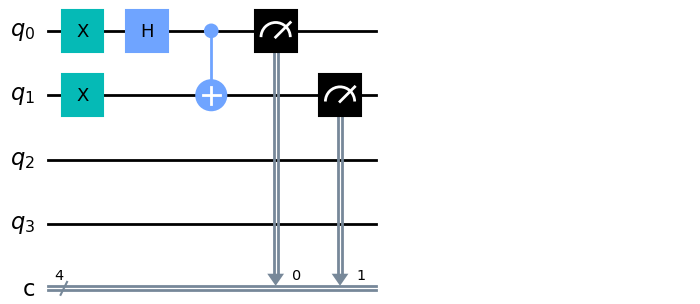
|
# Simulate the circuit
simulator = Aer.get_backend('qasm_simulator')
job = execute(circuit, simulator, shots=10000)
result = job.result()
# Get counts and plot the histogram
counts = result.get_counts(circuit)
plot_histogram(counts)
|
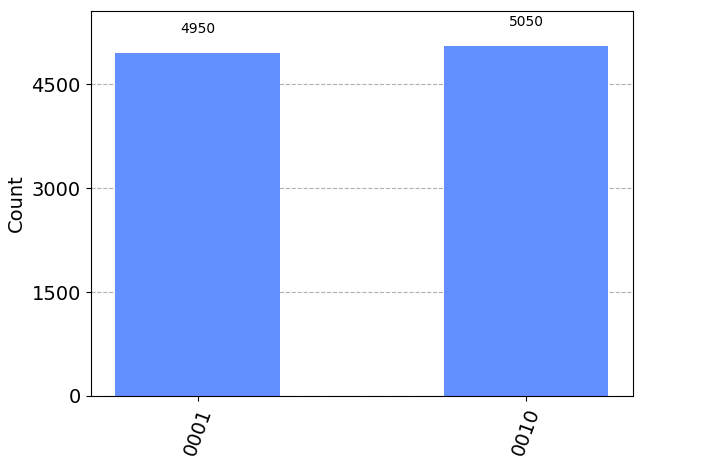
|
HH-CNOT with |0> and |0>
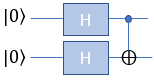
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
from qiskit.visualization import plot_histogram
from numpy import pi
qreg_q = QuantumRegister(4, 'q')
creg_c = ClassicalRegister(4, 'c')
circuit = QuantumCircuit(qreg_q, creg_c)
circuit.h(qreg_q[0])
circuit.h(qreg_q[1])
circuit.cx(qreg_q[0], qreg_q[1])
circuit.measure(qreg_q[0], creg_c[0])
circuit.measure(qreg_q[1], creg_c[1])
circuit.draw('mpl')
|
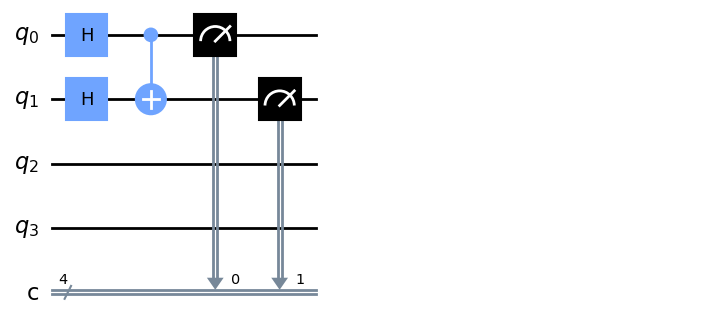
|
simulator = Aer.get_backend('qasm_simulator')
job = execute(circuit, simulator, shots=10000)
result = job.result()
# Get counts and plot the histogram
counts = result.get_counts(circuit)
plot_histogram(counts)
|
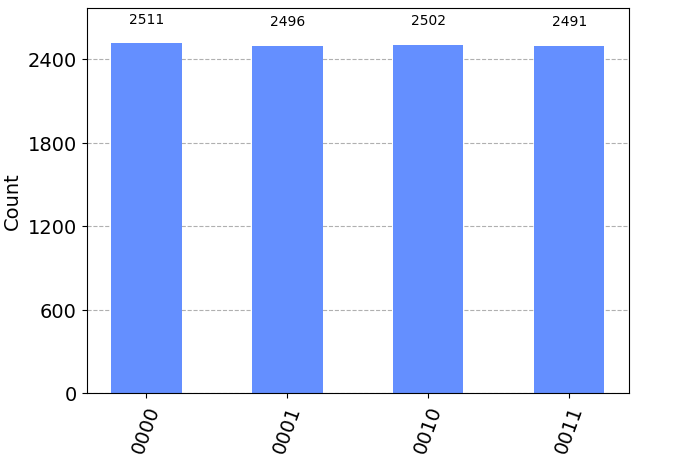
|
HH-CNOT with |1> and |1>
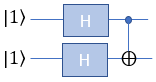
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
from qiskit.visualization import plot_histogram
from numpy import pi
qreg_q = QuantumRegister(4, 'q')
creg_c = ClassicalRegister(4, 'c')
circuit = QuantumCircuit(qreg_q, creg_c)
circuit.x(qreg_q[0])
circuit.h(qreg_q[0])
circuit.x(qreg_q[1])
circuit.h(qreg_q[1])
circuit.cx(qreg_q[0], qreg_q[1])
circuit.measure(qreg_q[0], creg_c[0])
circuit.measure(qreg_q[1], creg_c[1])
circuit.draw('mpl')
|
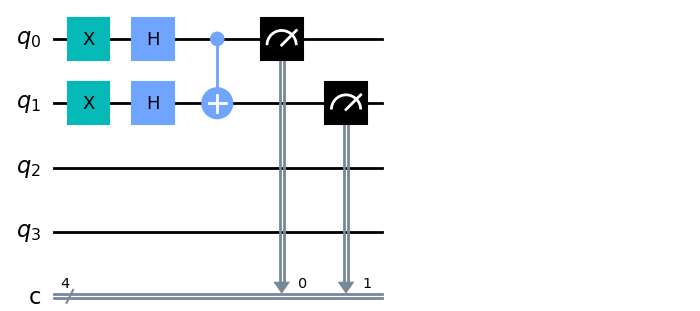
|
simulator = Aer.get_backend('qasm_simulator')
job = execute(circuit, simulator, shots=10000)
result = job.result()
# Get counts and plot the histogram
counts = result.get_counts(circuit)
plot_histogram(counts)
|
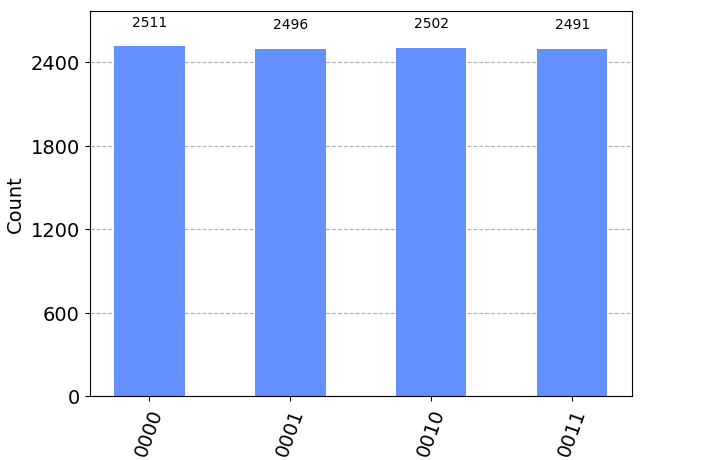
|
nnH-Toffoli-|111>
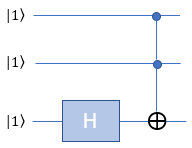
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, Aer, execute
from qiskit.visualization import plot_histogram
from numpy import pi
import matplotlib.pyplot as plt
qreg_q = QuantumRegister(4, 'q')
creg_c = ClassicalRegister(4, 'c')
circuit = QuantumCircuit(qreg_q, creg_c)
circuit.x(qreg_q[0])
circuit.x(qreg_q[1])
circuit.x(qreg_q[2])
circuit.h(qreg_q[2])
circuit.ccx(qreg_q[0], qreg_q[1], qreg_q[2])
circuit.measure(qreg_q[0], creg_c[0])
circuit.measure(qreg_q[1], creg_c[1])
circuit.measure(qreg_q[2], creg_c[2])
circuit.draw('mpl')
|
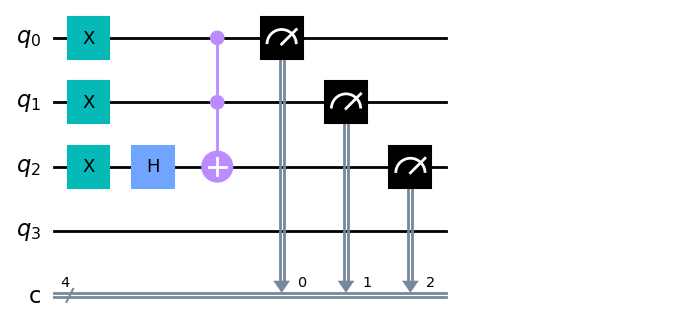
|
simulator = Aer.get_backend('qasm_simulator')
job = execute(circuit, simulator, shots=10000)
result = job.result()
# Get counts and plot the histogram
counts = result.get_counts(circuit)
plot_histogram(counts)
|
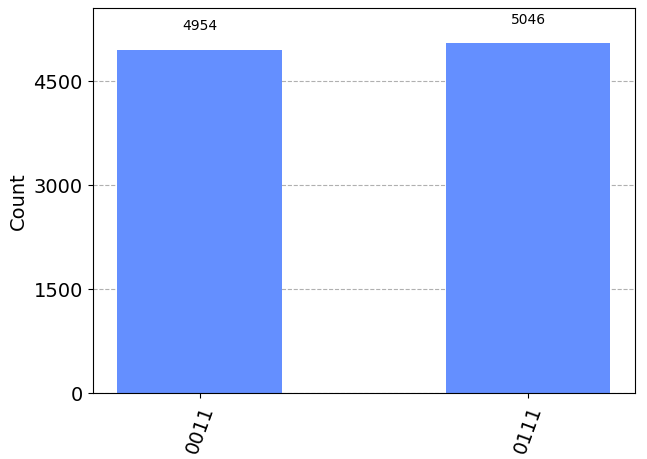
|
Hnn-Toffoli-|111>
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, Aer, execute
from qiskit.visualization import plot_histogram
from numpy import pi
import matplotlib.pyplot as plt
qreg_q = QuantumRegister(4, 'q')
creg_c = ClassicalRegister(4, 'c')
circuit = QuantumCircuit(qreg_q, creg_c)
circuit.x(qreg_q[0])
circuit.h(qreg_q[0])
circuit.x(qreg_q[1])
circuit.x(qreg_q[2])
circuit.ccx(qreg_q[0], qreg_q[1], qreg_q[2])
circuit.measure(qreg_q[0], creg_c[0])
circuit.measure(qreg_q[1], creg_c[1])
circuit.measure(qreg_q[2], creg_c[2])
circuit.draw('mpl')
|
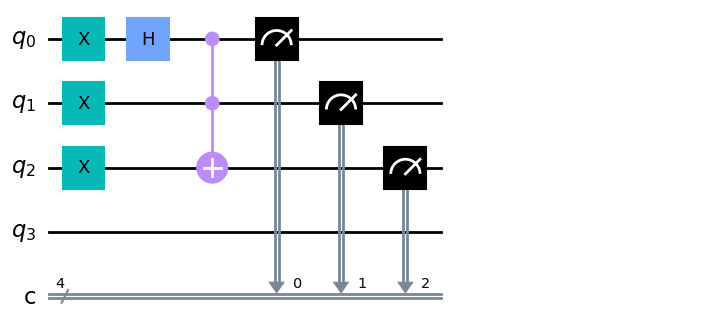
|
simulator = Aer.get_backend('qasm_simulator')
job = execute(circuit, simulator, shots=10000)
result = job.result()
# Get counts and plot the histogram
counts = result.get_counts(circuit)
plot_histogram(counts)
|
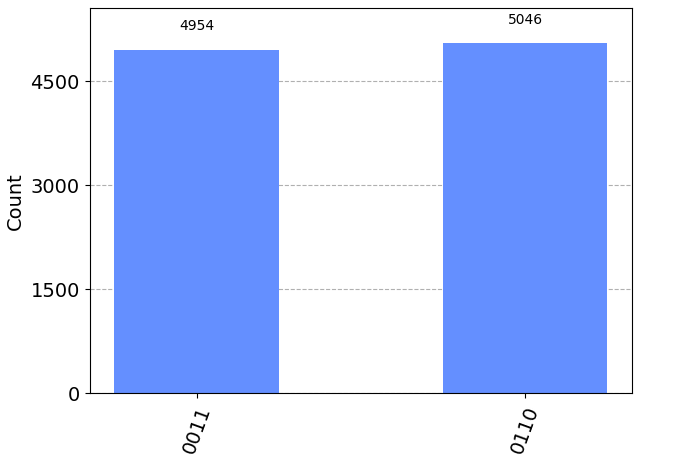
|
HHn-Toffoli-|111>
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, Aer, execute
from qiskit.visualization import plot_histogram
from numpy import pi
import matplotlib.pyplot as plt
qreg_q = QuantumRegister(4, 'q')
creg_c = ClassicalRegister(4, 'c')
circuit = QuantumCircuit(qreg_q, creg_c)
circuit.x(qreg_q[0])
circuit.h(qreg_q[0])
circuit.x(qreg_q[1])
circuit.h(qreg_q[1])
circuit.x(qreg_q[2])
circuit.ccx(qreg_q[0], qreg_q[1], qreg_q[2])
circuit.measure(qreg_q[0], creg_c[0])
circuit.measure(qreg_q[1], creg_c[1])
circuit.measure(qreg_q[2], creg_c[2])
circuit.draw('mpl')
|
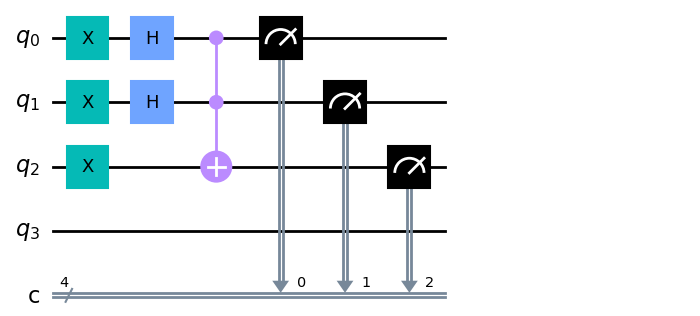
|
simulator = Aer.get_backend('qasm_simulator')
job = execute(circuit, simulator, shots=10000)
result = job.result()
# Get counts and plot the histogram
counts = result.get_counts(circuit)
plot_histogram(counts)
|
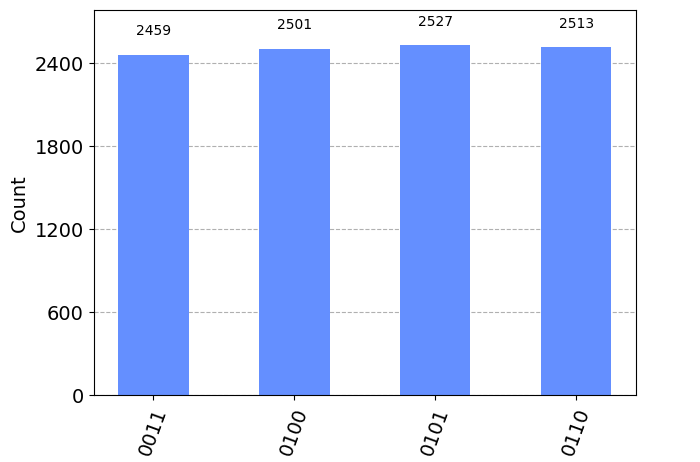
|
HHH-Toffoli-|111>
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, Aer, execute
from qiskit.visualization import plot_histogram
from numpy import pi
import matplotlib.pyplot as plt
qreg_q = QuantumRegister(4, 'q')
creg_c = ClassicalRegister(4, 'c')
circuit = QuantumCircuit(qreg_q, creg_c)
circuit.x(qreg_q[0])
circuit.h(qreg_q[0])
circuit.x(qreg_q[1])
circuit.h(qreg_q[1])
circuit.x(qreg_q[2])
circuit.h(qreg_q[2])
circuit.ccx(qreg_q[0], qreg_q[1], qreg_q[2])
circuit.measure(qreg_q[0], creg_c[0])
circuit.measure(qreg_q[1], creg_c[1])
circuit.measure(qreg_q[2], creg_c[2])
circuit.draw('mpl')
|
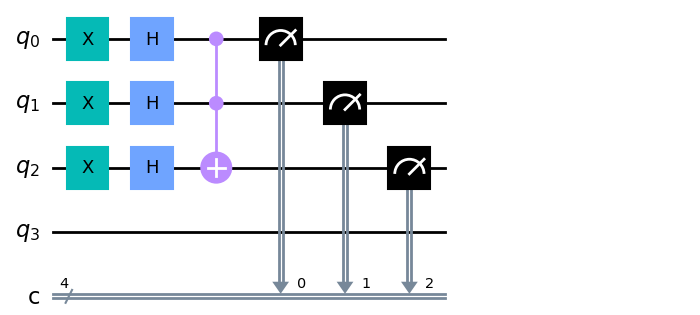
|
simulator = Aer.get_backend('qasm_simulator')
job = execute(circuit, simulator, shots=10000)
result = job.result()
# Get counts and plot the histogram
counts = result.get_counts(circuit)
plot_histogram(counts)
|
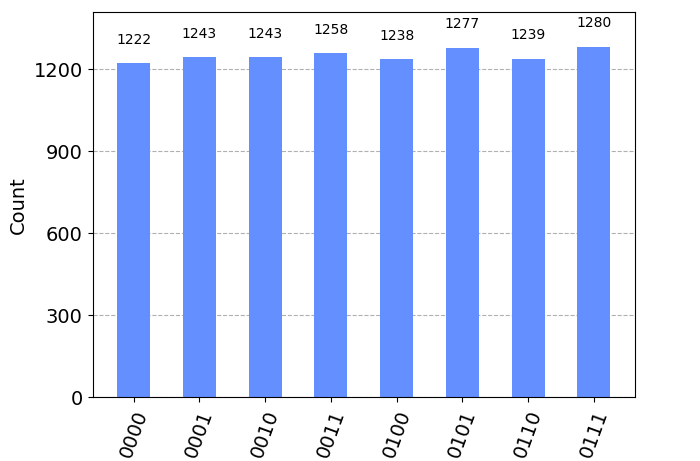
|
YouTube
|
|